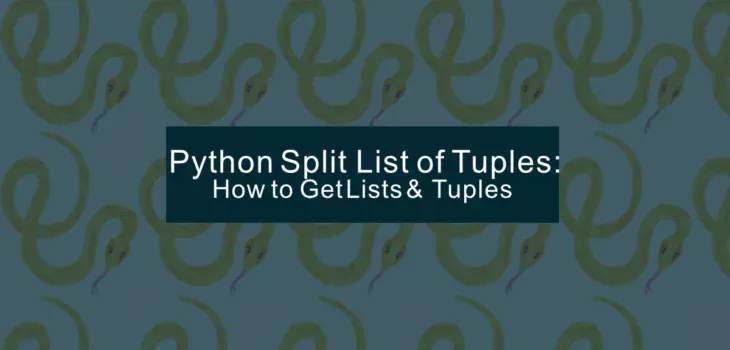
Python Split List of Tuples: How to Get Lists…
Python split list of tuples: If you’ve ever needed to work with data in tuples and wonder how to manipulate and extract information from them efficiently, you’re in the right place. In a previous post, we looked at how to remove punctuation from a string in Python, an essential skill for data wrangling and text processing.
Tuple splitting is a fundamental operation in Python programming with widespread relevance. Understanding how to split tuples opens doors to a variety of real-world applications. Splitting tuples is a valuable skill, whether you’re dealing with data processing, data analysis, or even creating more readable and maintainable code.

In the following sections, you will learn the ins and outs of tuple splitting. We’ll start with the basics, including how to perform simple tuple splitting using tuple unpacking. Then, we’ll dive deeper into more advanced techniques. You will discover how to split tuples based on specific conditions, use list comprehensions to streamline the process, and even create custom functions for intricate splitting scenarios.
By the end of this blog post, you will have a solid grasp of the techniques for splitting tuples, allowing you to extract and manipulate data efficiently in Python. You’ll be equipped with the knowledge to tackle a wide range of programming tasks, making your code more robust and adaptable. So, let’s begin our journey into Python tuple splitting.
Table of Contents
How to Split a List of Tuples in Python: Basic Tuple Splitting
Understanding how to split a list of tuples in Python programming is a foundational skill that can significantly enhance your data processing capabilities. In this section, we will delve into the basics of tuple splitting, focusing on the fundamental concept of tuple unpacking and the utility of the zip
function. By the end of this section, you’ll have the essential knowledge to perform basic tuple splitting and a grasp of when it is most useful.
1. The Fundamental Concept of Tuple Splitting: Tuple Unpacking
At its core, tuple splitting involves extracting elements from tuples and distributing them into separate lists. This process is achieved through a mechanism known as “tuple unpacking.” Python allows you to unpack the elements of a tuple and assign them to variables. For instance, consider a list of (name, age) tuples:
people = [('Alice', 25), ('Bob', 30), ('Charlie', 22)]
To use Pyton to split this list into two separate tuples for names and ages, you can employ tuple unpacking:
names, ages = zip(*people)
In this example, the zip
function, in combination with the *
operator, efficiently unpacks the tuples into two distinct lists, names
and ages
.
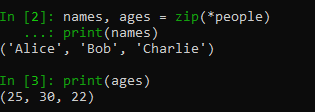
2. A Simple Example: Splitting a List of Tuples
Let’s illustrate this concept with a straightforward example. Consider the following list of tuples containing (item, quantity) pairs:
shopping_list = [('apples', 5), ('bananas', 3), ('cherries', 2)]
By applying tuple unpacking, you can create two separate lists for items and quantities:
items, quantities = zip(*shopping_list)
This results in the items
list containing the item names (‘apples’, ‘bananas’, ‘cherries’) and the quantities
list containing the corresponding quantities (5, 3, 2).
3. Using the zip
Function
In addition to tuple unpacking, Python offers the zip
function for tuple splitting. The zip
function combines multiple sequences into tuples, making it a useful tool. The example above demonstrates how zip can be applied to basic tuple splitting. It streamlines the process and is particularly handy when working with longer lists of tuples.
4. Use Cases of Basic Tuple Splitting
Basic tuple splitting is valuable in various scenarios. It simplifies tasks such as data transformation, extraction, and manipulation. For example, you might have a list of (x, y) tuples representing coordinates and need to extract all x-values and y-values separately for further analysis. Basic tuple splitting makes this task quick and efficient.
In summary, mastering basic tuple splitting is essential for any Python programmer. It is a fundamental operation that opens doors to more advanced techniques and is indispensable in various real-world applications, from data analysis to creating more organized and readable code.
Python Split List of Tuples: Splitting Based on a Specific Criterion
When working with lists of tuples in Python, it’s often necessary to split them based on specific criteria or conditions. This section will walk you through the process of achieving this, demonstrating the use of if statements and providing real-world examples to illustrate the versatility of this method.
Explaining the Process
To split a list of tuples based on a specific criterion, you can employ conditional statements to categorize and separate the tuples into distinct lists. Let’s delve into this approach using a real-world example.
Python Separating tuples containging Individuals Based on Their Age
Imagine you have a list of people with their respective ages:
people = [('Alice', 25), ('Bob', 30), ('Charlie', 22), ('David', 35)]
We want to split this list into two categories: ‘young’ and ‘old’ individuals, where the criterion for categorization is age. We can achieve this with if statements:
young, old = [], [] for name, age in people: if age < 30: young.append((name, age)) else: old.append((name, age))
In this example, we iterate through the Python list of tuples and use if statements to determine whether an individual is ‘young’ or ‘old’ based on their age. We then add them to the respective lists. Effectivley, we splitted the list of tuples into two lists.
Versatility for Custom Splitting
This method’s versatility extends beyond age-based splitting. You can apply it to various scenarios, customizing the condition to meet your specific requirements. Whether you need to categorize data, filter records, or organize information based on any criteria, this approach provides a powerful and adaptable solution.
By understanding how to split tuples based on specific conditions, you gain the flexibility to tailor your Python programs to a wide range of real-world applications.
Python Split List of Tuples: Splitting with List Comprehensions
List comprehensions offer a concise and powerful method for splitting a list of tuples in Python. In this section, we will introduce list comprehensions as an efficient approach to tuple splitting and provide examples demonstrating their use in categorizing tuples based on conditions. We will also discuss the advantages of list comprehensions, including improved code readability and brevity.
Introducing List Comprehensions
List comprehensions provide a succinct way to create new lists by applying an expression to each item in an existing iterable (such as a list or tuple). This makes them a valuable tool for tuple splitting based on conditions.
Examples of List Comprehensions for Tuple Splitting
Let’s illustrate the power of list comprehensions with examples. Consider a list of (index, number) tuples:
numbers = [(1, 42), (2, 17), (3, 98), (4, 31)]
We can use list comprehensions to efficiently split these tuples into two lists, one for even numbers and another for odd numbers:
even = [(idx, num) for idx, num in numbers if num % 2 == 0] odd = [(idx, num) for idx, num in numbers if num % 2 != 0]
These list comprehensions are concise, readable, and allow us to categorize tuples based on a specific condition with ease.
Advantages of List Comprehensions
List comprehensions offer several advantages when compared to traditional loops. They enhance code readability by expressing the operation concisely and directly. Additionally, list comprehensions often result in more compact and efficient code, reducing the need for verbose looping constructs.
By utilizing list comprehensions for tuple splitting, you can make your Python code more elegant and maintainable while achieving the desired functionality.
Python Split List of Tuples: Splitting Into Key-Value Pairs
Splitting a list of key-value tuples into two separate lists, one for keys and another for values, is a common task in data processing with Python. This section will explore this technique, explaining how to perform the split and providing practical examples to illustrate the process. We will also emphasize the usefulness of this method in various data-processing scenarios.
Explaining the Splitting Process
When you have a list of key-value tuples, the goal is to separate the keys and values into distinct lists. This can be achieved through tuple unpacking, much like our previous methods. The keys are unpacked into one list, while the values are unpacked into another.
Practical Examples of Key-Value Splitting Tuples
Let’s demonstrate the process with a practical example. Consider a list of (key, value) tuples:
data = [('name', 'Alice'), ('age', 25), ('city', 'New York')]
You can efficiently split this list into two lists, one for keys and another for values:
keys, values = zip(*data)
In this example, the ‘keys’ list will contain the key names (‘name’, ‘age’, ‘city’), and the ‘values’ list will contain the corresponding values (‘Alice’, 25, ‘New York’).
Usefulness in Data Processing
Splitting key-value tuples is a valuable technique when working with structured data, configuration settings, or any scenario where you need to separate metadata from actual values. It simplifies the process of extracting and manipulating specific information, allowing you to work with data more effectively.
Whether you’re dealing with dictionaries, configuration files, or database records, understanding how to split key-value tuples can streamline your data processing workflows and contribute to more organized and efficient Python code.
Python Split List of Tuples: Advanced Splitting with Custom Functions
While basic tuple splitting methods serve common scenarios, there are instances where more complex splitting criteria are needed. In this section, we will delve into such scenarios and introduce the concept of creating custom functions for splitting lists of tuples in Python. We’ll provide a practical example of custom function creation for splitting financial transactions, emphasizing how these functions offer flexibility and adaptability.
Complex Splitting Criteria in Python
In real-world data processing, you may encounter situations that demand more intricate splitting logic. Standard methods may not suffice for cases where data is structured in unique ways or requires custom categorization based on specific attributes. This is where custom functions become invaluable.
Introducing Custom Functions for Tuple Splitting
Custom functions are Python functions designed to handle specific tuple splitting requirements. They allow you to define precise criteria for separating data in a manner that suits your particular application. These functions are essential when you need tailored solutions for complex splitting tasks.
Example: Custom Function for Splitting Financial Transactions
Let’s consider an example that requires custom tuple splitting. You have a list of financial transactions, each represented as (category, amount) tuples. To distinguish income from expenses, you can create a custom function:
def split_by_category(data, category): return [item for item in data if item[0] == category]
In this case, you can call the custom function ‘split_by_category’ to categorize transactions as income or expenses. This illustrates how custom functions can handle specialized tasks and simplify complex tuple splitting.
Flexibility and Adaptability
Custom functions offer unparalleled flexibility and adaptability in tackling the most challenging tuple splitting scenarios. By tailoring functions to the specific needs of your Python code, you can efficiently manage data in ways that may not be achievable with standard methods.
Whether you’re working with financial data, multi-dimensional datasets, or any data structure with unique characteristics, understanding how to create and use custom functions for splitting lists of tuples in Python is a valuable skill that enhances your programming capabilities.
Splitting Lists of Nested Tuples in Python
While basic tuple splitting techniques can handle many scenarios, you may encounter situations where tuples within tuples need to be split. This section explores the complexities of handling nested tuples and how to effectively extract desired information from them, emphasizing the significance of understanding tuple structures when dealing with such cases.
Dealing with Nested Tuples
In data processing and Python programming, you may encounter nested tuples, where each tuple contains sub-tuples. This presents a unique challenge when you need to split the inner tuples or access specific elements. Understanding how to navigate and split nested tuples is essential for comprehensive data manipulation.
Examples of Handling Nested Tuples
Consider a scenario where you have a list of tuples, and each tuple contains sub-tuples. For instance:
data = [(('Alice', 'Smith'), (25, 'New York')), (('Bob', 'Johnson'), (30, 'Los Angeles'))]
Handling this structure requires accessing and splitting the inner sub-tuples. You can use nested tuple unpacking to extract the desired information:
for (name, surname), (age, city) in data: print(f"Name: {name} {surname}, Age: {age}, City: {city}")
By understanding the structure and employing nested tuple unpacking, you can efficiently access and manipulate the data within nested tuples.
Importance of Understanding Tuple Structure
When dealing with nested tuples, comprehending the tuple structure is paramount. It allows you to access and split the data accurately. Without a solid grasp of the tuple hierarchy, you may encounter difficulties navigating and extracting the desired information. Therefore, it’s crucial to master this skill when working with complex data structures in Python.
Understanding how to handle nested tuples is essential to splitting lists of tuples in Python, ensuring that you can effectively work with data organized in multiple layers.
Conclusion
In this journey through Python tuple splitting, you’ve learned a variety of methods to manipulate and extract information from lists of tuples efficiently. Each technique offers a valuable approach to tackling different data processing scenarios, from basic tuple unpacking to advanced custom functions.
The practical significance of tuple splitting in Python cannot be understated. It’s a fundamental skill that empowers you to work with data more effectively, enhancing code readability and efficiency. Whether you’re dealing with data wrangling, analysis, or data transformation, these techniques are indispensable.
We encourage you to experiment with the methods discussed and apply them to your own Python projects. By mastering the art of tuple splitting, you’ll become a more versatile and capable Python programmer, equipped to excel in data wrangling, analysis, and beyond.
For more insights into data wrangling in Python, you can explore our dedicated post on Data Wrangling in Python, where we delve deeper into the techniques and tools for cleaning, transforming, and preparing data for analysis.